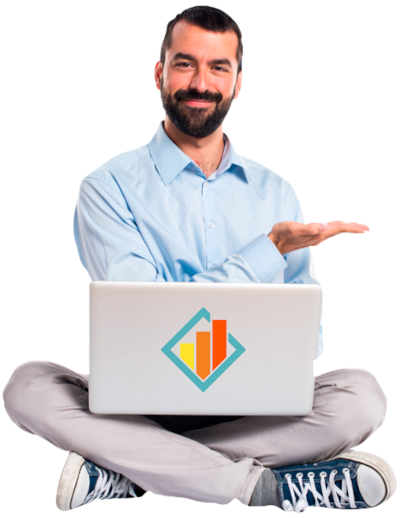
Services
Notre Processus
Nous nous concentrons sur vos objectifs pour élaborer des solutions de conception sur mesure pour votre projet.
Étape 1
- Nous prenons le temps de comprendre vos objectifs, défis et opportunités. Ensemble, nous définissons des objectifs et élaborons une stratégie.
Étape 2
- Nous démarrons avec des délais clairs, des jalons et des priorités. Notre équipe effectue adapte sa stratégie à votre audience et à votre secteur.
Étape 3
- En collaboration avec vous, nous affinons les idées pour les transformer en concepts concrets. Cette phase équilibre créativité avec objectif SMART .
Étape 4
- Nos designers créent des prototypes centrés sur l’utilisateur, en veillant à la fonctionnalité et à la cohérence avec l’identité de votre marque.
Étape 5
- Grâce à des tests rigoureux, nous validons le design en conditions réelles. Chaque détail est affiné avec soin avant le lancement.
Étape 6
- Nous vous fournissons une documentation pour garantir une transition fluide et restons disponibles pour un accompagnement.
FAQ
Vous avez d’autres questions ou vous êtes simplement curieux de découvrir les possibilités futures ?
1. Aidez-vous à la refonte des produits ?
Oui, nous sommes spécialisés dans la refonte de produits. Que ce soit pour un nouveau look, une meilleure expérience utilisateur ou de nouvelles fonctionnalités, nous pouvons vous accompagner. Notre équipe réalise des recherches approfondies et travaille avec vous pour créer une stratégie de refonte sur mesure.
2. Combien de temps dure l’ensemble du processus de conception ?
3. Comment assurez-vous une livraison dans les délais ?
4. Comment maintenez-vous la communication pendant le projet ?
5. Vos conceptions sont-elles optimisées pour tous les appareils ?
6. Travaillez-vous uniquement sur la conception de produits numériques ?
7. Avec quel type d'entreprises travaillez-vous ?
Trame Méthodologique de Gestion de Projet : Guide Complet 2025
La gestion de projet nécessite une méthode rigoureuse et structurée pour garantir la réussite des objectifs fixés, tout en respectant les contraintes de temps, de budget et de ressources. Dans un environnement professionnel de plus en plus complexe, 90% des projets...
Guide pratique : – SEO Technique
Bienvenue dans cette page dédiée au SEO technique. Dans ce contenu approfondi, vous découvrirez : Le crawling et l'indexation optimisés Les sitemaps XML et leur exploitation stratégique Comment résoudre les problèmes de contenu dupliqué L'implémentation avancée des...
Guide pratique : – SEO On-Page
Maîtriser le SEO on-page peut avoir un impact considérable sur vos classements, votre trafic et vos conversions. Dans cette page je vous présente : Créer un contenu adapté à la fois aux moteurs de recherche et aux utilisateurs Concevoir des URLs optimisées pour le...